Lombok - @XArgsConstructor Advanced
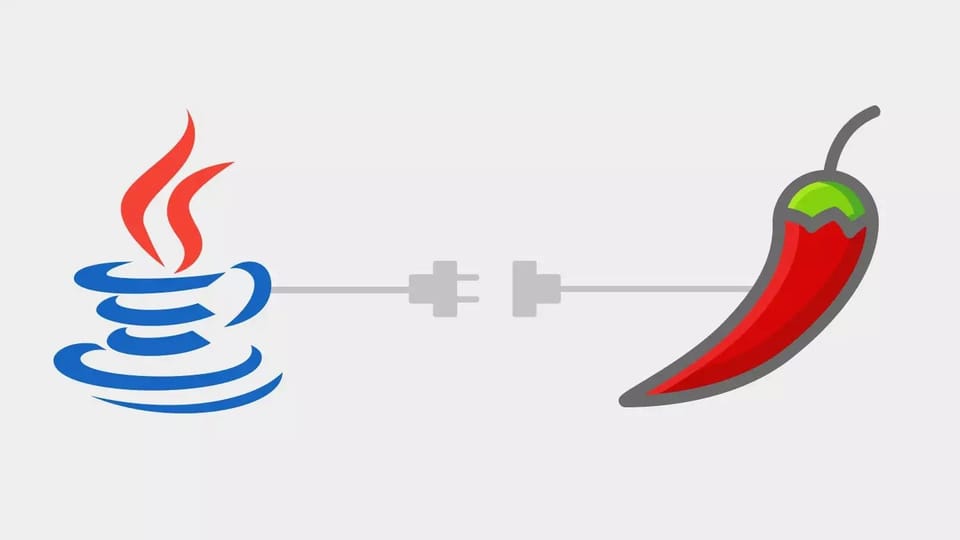
Static factory design pattern with Lombok
One of the great things about Lombok is the fact that you can generate code which complies to the best practices in software development since Lombok was written by some of the brightest minds in this field.
An example is the possibility of generating classes which include a static of method to create instances of a specific class.
Let's look at the example below:
package nl.emsnsoftware.entity;
import lombok.NoArgsConstructor;
import java.time.LocalDate;
@NoArgsConstructor(staticName="of")
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
int count;
}
By adding the staticName="of" attribute to the @NoArgsConstructor annotation, a new form of generation will be applied. Let's check the result and discuss the differences:
package nl.emsnsoftware.entity;
import java.time.LocalDate;
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
int count;
private Car() {
}
public static Car of() {
return new Car();
}
}
In the previous code snippet, we can notice that a private constructor was generated instead of a public constructor. Additionally a static of method was generated in order to be able to create new instances of the Car class.
Enum constructors with Lombok
All 3 constructor annotations @NoArgsConstructor, @RequiredArgsConstructor and @AllArgsConstructor can be used on an enum definition.
Here is an example. Below the .java code of the Color enum.
package nl.emsnsoftware.enums;
import lombok.AllArgsConstructor;
@AllArgsConstructor()
public enum Color {
RED,
GREEN,
BLUE,
}
The color.class compiled code will look like this:
package nl.emsnsoftware.enums;
public enum Color {
RED,
GREEN,
BLUE;
private Color() {
}
}
One main difference by using these annotations on Enum definitions, is the fact that the generated constructor has a private access modifier. That's because non-private constructors aren't legal in enums.
Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog.