Lombok - @Value
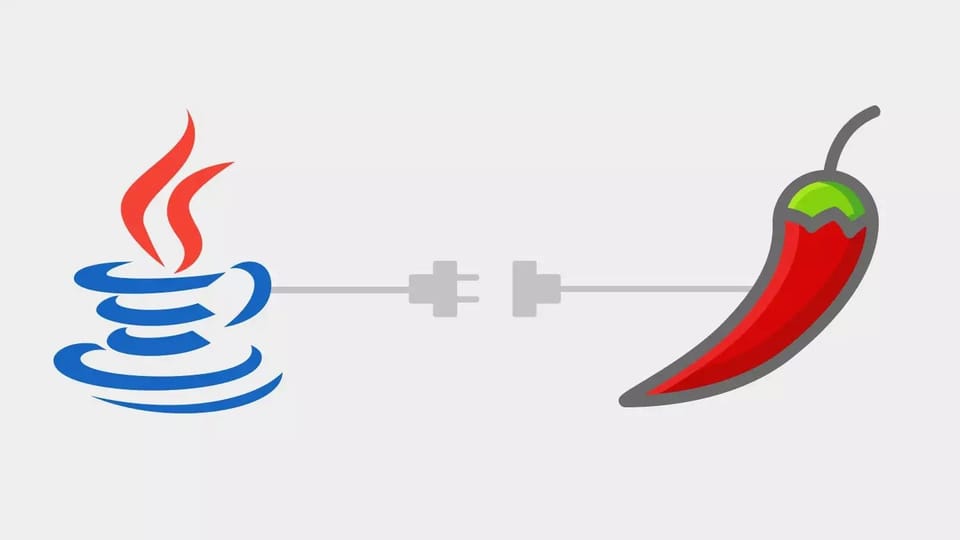
@Value is the immutable variant of @Data. All fields are made private and final by default, and setters are not generated. The class itself is also made final by default.
If you have been using a new version of Java(Java 16+) then you may have used Java Records to achieve immutability. If you are not familier with Records, they are classes that act as transparent carriers for immutable data. While @Value and Record look similair, immutable data, there are fundamentel differences between them.
The most important one is that the sole purpose of @Value is to remove boilerplate code, while Records core semantic is data that's immutable and transparently accessible. So make sure to choose wisely between them.
Ok, time for an example..
package nl.emsnsoftware.entity;
import lombok.*;
import java.time.LocalDate;
@Value
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
int count;
}
And the generated .class file:
package nl.emsnsoftware.entity;
import java.time.LocalDate;
public final class Car {
private final String licensePlate;
private final String brand;
private final LocalDate manufacturingDate;
private final boolean inUse;
private final int count;
public Car(String licensePlate, String brand, LocalDate manufacturingDate, boolean inUse, int count) {
this.licensePlate = licensePlate;
this.brand = brand;
this.manufacturingDate = manufacturingDate;
this.inUse = inUse;
this.count = count;
}
public String getLicensePlate() {
return this.licensePlate;
}
public String getBrand() {
return this.brand;
}
public LocalDate getManufacturingDate() {
return this.manufacturingDate;
}
public boolean isInUse() {
return this.inUse;
}
public int getCount() {
return this.count;
}
public boolean equals(Object o) {
if (o == this) {
return true;
} else if (!(o instanceof Car)) {
return false;
} else {
Car other = (Car)o;
if (this.isInUse() != other.isInUse()) {
return false;
} else if (this.getCount() != other.getCount()) {
return false;
} else {
label49: {
Object this$licensePlate = this.getLicensePlate();
Object other$licensePlate = other.getLicensePlate();
if (this$licensePlate == null) {
if (other$licensePlate == null) {
break label49;
}
} else if (this$licensePlate.equals(other$licensePlate)) {
break label49;
}
return false;
}
Object this$brand = this.getBrand();
Object other$brand = other.getBrand();
if (this$brand == null) {
if (other$brand != null) {
return false;
}
} else if (!this$brand.equals(other$brand)) {
return false;
}
Object this$manufacturingDate = this.getManufacturingDate();
Object other$manufacturingDate = other.getManufacturingDate();
if (this$manufacturingDate == null) {
if (other$manufacturingDate != null) {
return false;
}
} else if (!this$manufacturingDate.equals(other$manufacturingDate)) {
return false;
}
return true;
}
}
}
public int hashCode() {
int PRIME = true;
int result = 1;
int result = result * 59 + (this.isInUse() ? 79 : 97);
result = result * 59 + this.getCount();
Object $licensePlate = this.getLicensePlate();
result = result * 59 + ($licensePlate == null ? 43 : $licensePlate.hashCode());
Object $brand = this.getBrand();
result = result * 59 + ($brand == null ? 43 : $brand.hashCode());
Object $manufacturingDate = this.getManufacturingDate();
result = result * 59 + ($manufacturingDate == null ? 43 : $manufacturingDate.hashCode());
return result;
}
public String toString() {
String var10000 = this.getLicensePlate();
return "Car(licensePlate=" + var10000 + ", brand=" + this.getBrand() + ", manufacturingDate=" + this.getManufacturingDate() + ", inUse=" + this.isInUse() + ", count=" + this.getCount() + ")";
}
}
That is a lot of boilerplate code which we did not have to write and was easily generated for us!
Shortly, @Value is shorthand for: final @ToString @EqualsAndHashCode @AllArgsConstructor @FieldDefaults(makeFinal = true, level = AccessLevel.PRIVATE) @Getter.
Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog.