Lombok - @ToString
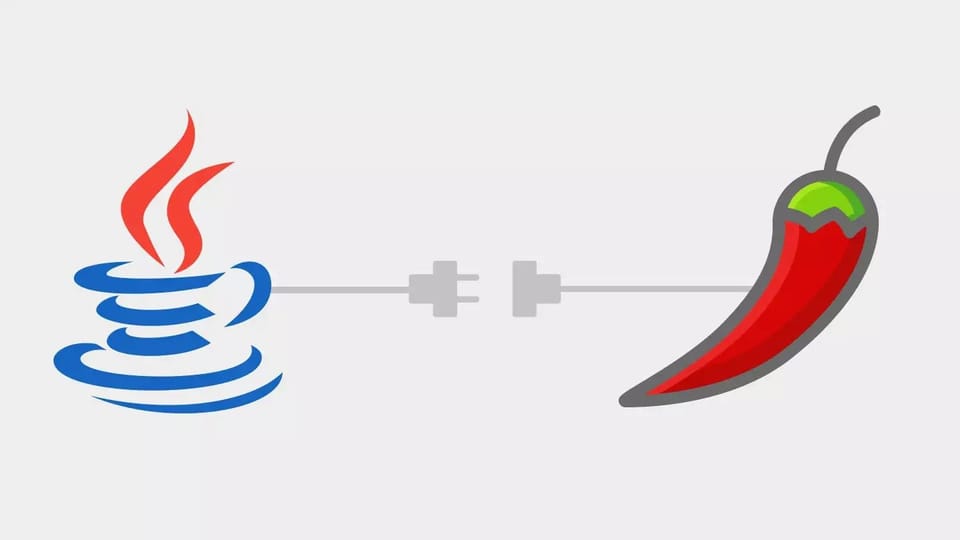
A class definition annotated with @ToString lets Lombok generate the toString() method implementation. By default, it'll print the class name, along with each field, in order and separated by commas.
Let's take a look at the following example which shows the basic usage of this annotation.
package nl.emsnsoftware.entity;
import lombok.*;
import java.time.LocalDate;
@NoArgsConstructor()
@ToString
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
static int count;
}
And the generated code:
package nl.emsnsoftware.entity;
import java.time.LocalDate;
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
static int count;
public Car() {
}
public String toString() {
return "Car(licensePlate=" + this.licensePlate + ", brand=" + this.brand + ", manufacturingDate=" + this.manufacturingDate + ", inUse=" + this.inUse + ")";
}
}
From this example, we can notice that Lombok generated the toString method including the name of the class plus all non-static fields of the class.
Now let's say we do not want to include all non-static fields in the toString method, what can we do? Lombok provides way for achiving this.
- By annotating these fields with @ToString.Exclude.
package nl.emsnsoftware.entity;
import lombok.*;
import java.time.LocalDate;
@NoArgsConstructor()
@ToString
public class Car {
String licensePlate;
String brand;
@ToString.Exclude
LocalDate manufacturingDate;
@ToString.Exclude
boolean inUse;
static int count;
}
package nl.emsnsoftware.entity;
import java.time.LocalDate;
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
static int count;
public Car() {
}
public String toString() {
return "Car(licensePlate=" + this.licensePlate + ", brand=" + this.brand + ")";
}
}
- Or adding @ToString(onlyExplicitlyIncluded = true) to the class definition and annotating the required fields with @ToString.Include.
package nl.emsnsoftware.entity;
import lombok.*;
import java.time.LocalDate;
@NoArgsConstructor()
@ToString(onlyExplicitlyIncluded = true)
public class Car {
@ToString.Include
String licensePlate;
@ToString.Include
String brand;
LocalDate manufacturingDate;
boolean inUse;
static int count;
}
package nl.emsnsoftware.entity;
import java.time.LocalDate;
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
static int count;
public Car() {
}
public String toString() {
return "Car(licensePlate=" + this.licensePlate + ", brand=" + this.brand + ")";
}
}
Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog.