Lombok - @RequiredArgsConstructor
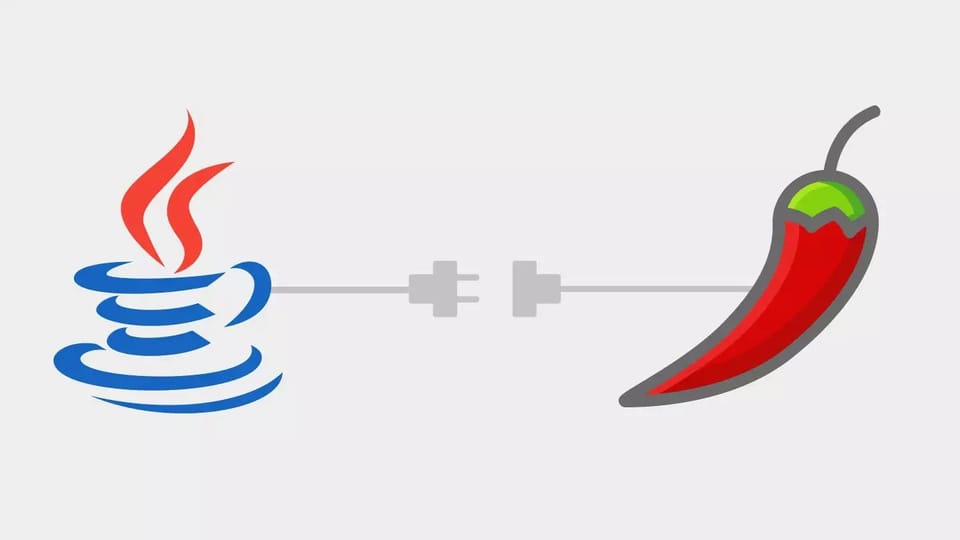
Let's move to another very popular annotation from the Lombok project, the @RequiredArgsConstructor. The @RequiredArgsConstructor will generate 1 parameter for each field that requires special handling. But wait a minute, what are field that requires special handling?
- All non-initialized final fields.
- All fields that are marked as @NonNull that aren't initialized where declared.
Let's take a look at some examples:
Here is the code for our Car class. What do you think the constructor will look like in the compiled code?
package nl.emsnsoftware.entity;
import lombok.RequiredArgsConstructor;
import java.time.LocalDate;
@RequiredArgsConstructor
public class Car {
final String licensePlate;
String brand;
LocalDate manufacturingDate;
final boolean inUse;
final int count;
}
After compiling the code by running mvn clean install, our car.class file will look like the following:
package nl.emsnsoftware.entity;
import java.time.LocalDate;
public class Car {
final String licensePlate;
String brand;
LocalDate manufacturingDate;
final boolean inUse;
final int count;
public Car(String licensePlate, boolean inUse, int count) {
this.licensePlate = licensePlate;
this.inUse = inUse;
this.count = count;
}
}
Please notice that the constructor was generated with parameters for final required field only and not for non-final fields such as brand and manufacturingDate.
Now let us look at an example where @NonNull annotation is used and check the difference. Here is the code for our modified Car class.
package nl.emsnsoftware.entity;
import lombok.NonNull;
import lombok.RequiredArgsConstructor;
import java.time.LocalDate;
@RequiredArgsConstructor
public class Car {
@NonNull
final String licensePlate;
String brand;
@NonNull
LocalDate manufacturingDate;
final boolean inUse;
final int count;
}
We have marked the licensePlate and manufacturingDate instance variables with @NonNull annotation. Lombok will in this case generate a constructor which will include an explicit null check for those fields. The constructor will throw a NullPointerException if any of the parameters intended for the fields marked with @NonNull contain null and the order of the parameters match the order in which the fields appear in your class.
package nl.emsnsoftware.entity;
import java.time.LocalDate;
import lombok.NonNull;
public class Car {
@NonNull
final String licensePlate;
String brand;
@NonNull
LocalDate manufacturingDate;
final boolean inUse;
final int count;
public Car(@NonNull String licensePlate, @NonNull LocalDate manufacturingDate, boolean inUse, int count) {
if (licensePlate == null) {
throw new NullPointerException("licensePlate is marked non-null but is null");
} else if (manufacturingDate == null) {
throw new NullPointerException("manufacturingDate is marked non-null but is null");
} else {
this.licensePlate = licensePlate;
this.manufacturingDate = manufacturingDate;
this.inUse = inUse;
this.count = count;
}
}
}
Looking at the last example, you can already see the power of Lombok. The compiled code is 2 times the size of the code we wrote using Lombok for one class. Imaging the effect of using Lombok in your production system where you have hundreds if not thousands classes.
Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog.