Lombok - @NonNull
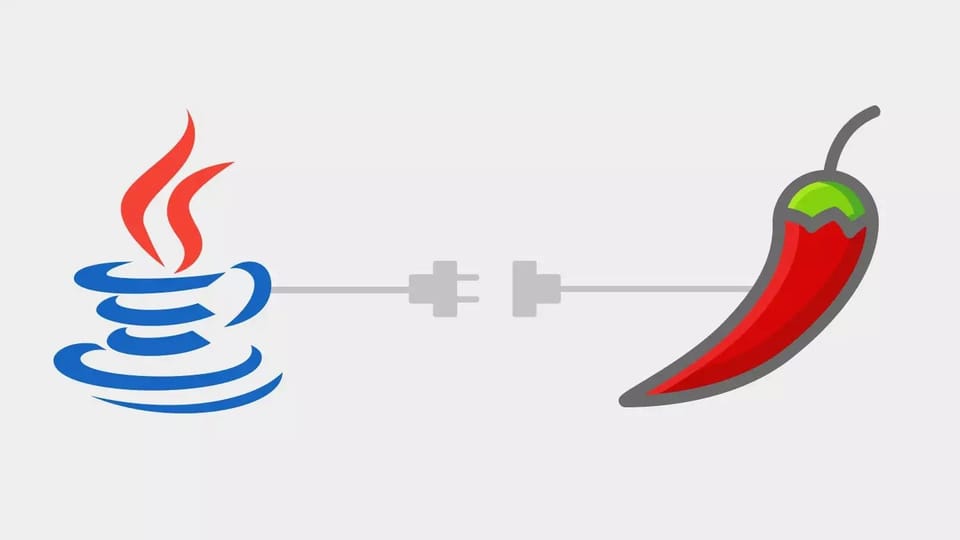
Writing Java code that does not perform the necessary checks for NullPointerException is one of the pitfalls of Java and the most common source of bugs. If you are wondering why, it's because NullPointerException is a RuntimeException, meaning at runtime no object is created and the compiler does not detect it beforehand.
That is why it is necessary to check for null values where you expect that null could be assigned to references. This is again a very repetitive work and can produce unreadable code base.
Lombok provides a solution for this problem. By using the @NotNull annotation, Lombok will generate a null-check statement for you.
Let's take a look at an example:
package nl.emsnsoftware.entity;
import lombok.*;
import java.time.LocalDate;
@AllArgsConstructor
public class Car {
@NonNull @Getter @Setter
String licensePlate;
String brand;
@NonNull
LocalDate manufacturingDate;
boolean inUse;
static int count;
}
And after compiling the code, we can see the following Car.class file:
package nl.emsnsoftware.entity;
import java.time.LocalDate;
import lombok.NonNull;
public class Car {
@NonNull
String licensePlate;
String brand;
@NonNull
LocalDate manufacturingDate;
boolean inUse;
static int count;
public Car(@NonNull String licensePlate, String brand, @NonNull LocalDate manufacturingDate, boolean inUse) {
if (licensePlate == null) {
throw new NullPointerException("licensePlate is marked non-null but is null");
} else if (manufacturingDate == null) {
throw new NullPointerException("manufacturingDate is marked non-null but is null");
} else {
this.licensePlate = licensePlate;
this.brand = brand;
this.manufacturingDate = manufacturingDate;
this.inUse = inUse;
}
}
@NonNull
public String getLicensePlate() {
return this.licensePlate;
}
public void setLicensePlate(@NonNull String licensePlate) {
if (licensePlate == null) {
throw new NullPointerException("licensePlate is marked non-null but is null");
} else {
this.licensePlate = licensePlate;
}
}
}
In the previous code snippet, we can notice 2 things:
- In the constructor, for fields annotated with @NotNull, a null check statement was generated which will throw a NullPointerException if the object is null.
- The setter method setLicensePlate() also includes a null check statement was generated which will throw a NullPointerException if the object is null.
OK, easy right? Let's try something.. what do you think will happen if I annotate the inUse field also with the @NotNull annotation? Let's check the result in the following code:
package nl.emsnsoftware.entity;
import java.time.LocalDate;
import lombok.NonNull;
public class Car {
@NonNull
String licensePlate;
String brand;
@NonNull
LocalDate manufacturingDate;
@NonNull
boolean inUse;
static int count;
public Car(@NonNull String licensePlate, String brand, @NonNull LocalDate manufacturingDate, @NonNull boolean inUse) {
if (licensePlate == null) {
throw new NullPointerException("licensePlate is marked non-null but is null");
} else if (manufacturingDate == null) {
throw new NullPointerException("manufacturingDate is marked non-null but is null");
} else {
this.licensePlate = licensePlate;
this.brand = brand;
this.manufacturingDate = manufacturingDate;
this.inUse = inUse;
}
}
@NonNull
public String getLicensePlate() {
return this.licensePlate;
}
public void setLicensePlate(@NonNull String licensePlate) {
if (licensePlate == null) {
throw new NullPointerException("licensePlate is marked non-null but is null");
} else {
this.licensePlate = licensePlate;
}
}
}
From the previous code snippet we can notice that Lombok did not generate a null check statement for inUse since it is of the primitive type boolean.
Sometimes you want to throw a different excpetion if the null check statements fails, for example the IllegalArgumentException, for that you need to create a file named lombok.config in the home directory of your project and add the following to it:
lombok.nonNull.exceptionType = IllegalArgumentException
Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog.