Lombok - @NoArgsConstructor
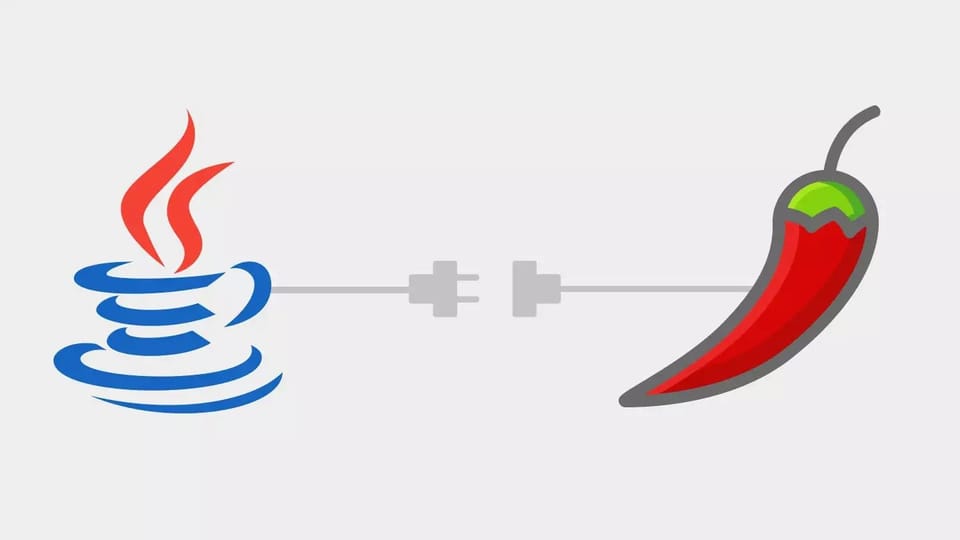
Let's start with the easies and one of the most used annotations from the Lombok project the @NoArgsConstructor. The @NoArgsConstructor will generate a constructor with no parameters. If this is not possible, a compiler error will result instead.
Let's take a look at some examples to cover the 2 previous scenario's.
package nl.emsnsoftware.entity;
import lombok.NoArgsConstructor;
import java.time.LocalDate;
@NoArgsConstructor
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
}
In the previous code snippet we have a plain Java class. Instead of writing a no arguments constructor for this class, we added the @NoArgsConstructor annotation. Let's see how the compiler will interpret this annotation by checking the bytecode .class file below.
package nl.emsnsoftware.entity;
import java.time.LocalDate;
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
public Car() {
}
}
Please notice in the previous code snippet how the compiled code includes the no arguments constructor that we intended to create by adding the lombok annotation.
What do you think will happen if we make one of the instance variables a final variable?
package nl.emsnsoftware.entity;
import lombok.NoArgsConstructor;
import java.time.LocalDate;
@NoArgsConstructor
public class Car {
final String licensePlate;
String brand;
LocalDate manufacturingDate;
}
The compiler will show an error (Variable 'licensePlate' might not have been initialized) since we are not assiging any value to the variable and no other constructors are available to initialize it.
Here is another question. I have added force = true to @NoArgsConstructor.
package nl.emsnsoftware.entity;
import lombok.NoArgsConstructor;
import java.time.LocalDate;
@NoArgsConstructor(force = true)
public class Car {
final String licensePlate;
String brand;
LocalDate manufacturingDate;
final boolean inUse;
final int count;
}
What do you think will the .class file look like?
The answer is as follows:
package nl.emsnsoftware.entity;
import java.time.LocalDate;
public class Car {
final String licensePlate = null;
String brand;
LocalDate manufacturingDate;
final boolean inUse = false;
final int count = 0;
public Car() {
}
}
Lombok initialized all final fields with 0/false/null based on the type of the variable.
At the end it is necessary to mention that this annotation is one of the most helpful Lombok annotations. Think how much easy your life would be when using it in combination with Hibernate and Spring-boot where some classes require to have a no-args constructor.
*Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog. *