Lombok - @Log
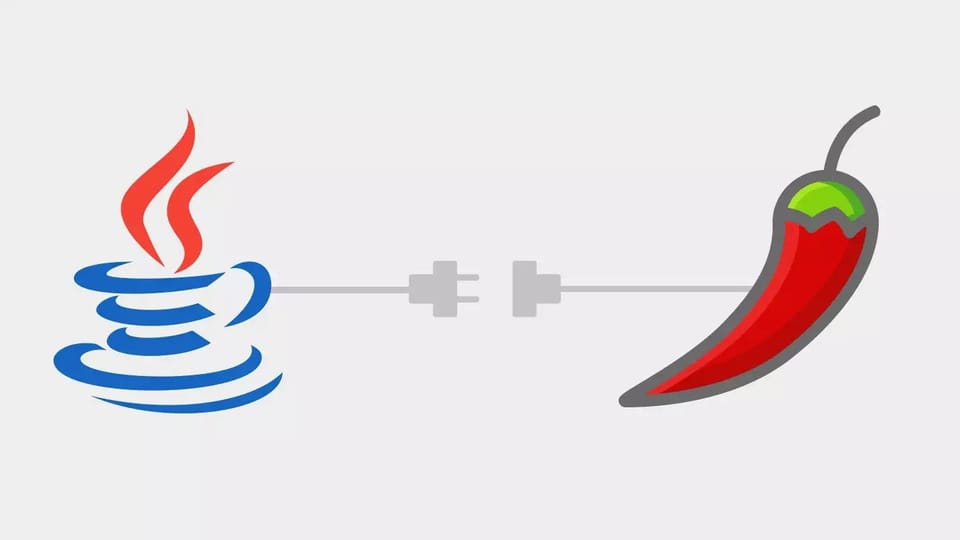
How many times did you have to write the following line of code? And how many times did you copy it from another class and made modifications to it?
private static final Logger log = LogManager.getLogger(Car.class);
With Lombok logging annotations you can easily use a logger in your classes without the need for explicitly declaring a logger.
Here's an example:
package nl.emsnsoftware;
import lombok.extern.java.Log;
@Log
public class App {
public static void main( String[] args ) {
log.info( "Hello World!" );
}
}
Because of the @Log annotation, we we able to use the log variable. Let's see how the .class file looks like after compilation.
package nl.emsnsoftware;
import java.util.logging.Logger;
public class App {
private static final Logger log = Logger.getLogger(App.class.getName());
public App() {
}
public static void main(String[] args) {
log.info("Hello World!");
}
}
As explained earlier, a class variable "log" declaration was generated because of the @Log annotation.
But wait a minutes, this is the default Java util Logger(java.util.logging.Logger)... what if you are using a Logger from a different framework like Log4J?
Lombok provides several annotations which support the major and most commonly used logging frameworks.
@CommonsLog
private static final org.apache.commons.logging.Log log = org.apache.commons.logging.LogFactory.getLog(Car.class);
@Flogger
private static final com.google.common.flogger.FluentLogger log = com.google.common.flogger.FluentLogger.forEnclosingClass();
@JBossLog
private static final org.jboss.logging.Logger log = org.jboss.logging.Logger.getLogger(Car.class);
@Log4j
private static final org.apache.log4j.Logger log = org.apache.log4j.Logger.getLogger(Car.class);
@Log4j2
private static final org.apache.logging.log4j.Logger log = org.apache.logging.log4j.LogManager.getLogger(Car.class);
@Slf4j
private static final org.slf4j.Logger log = org.slf4j.LoggerFactory.getLogger(Car.class);
@XSlf4j
private static final org.slf4j.ext.XLogger log = org.slf4j.ext.XLoggerFactory.getXLogger(Car.class);
You can even use @CustomLog annotation to declare a custom logger.
@CustomLog
private static final com.foo.your.Logger log = com.foo.your.LoggerFactory.createYourLogger(Car.class);
For this option you need to add a configuration to your lombok.config file to specify what @CustomLog should do.For example:lombok.log.custom.declaration = com.foo.your.Logger com.foo.your.LoggerFactory.createYourLog(TYPE)
Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog.