Lombok - @Getter/@Setter
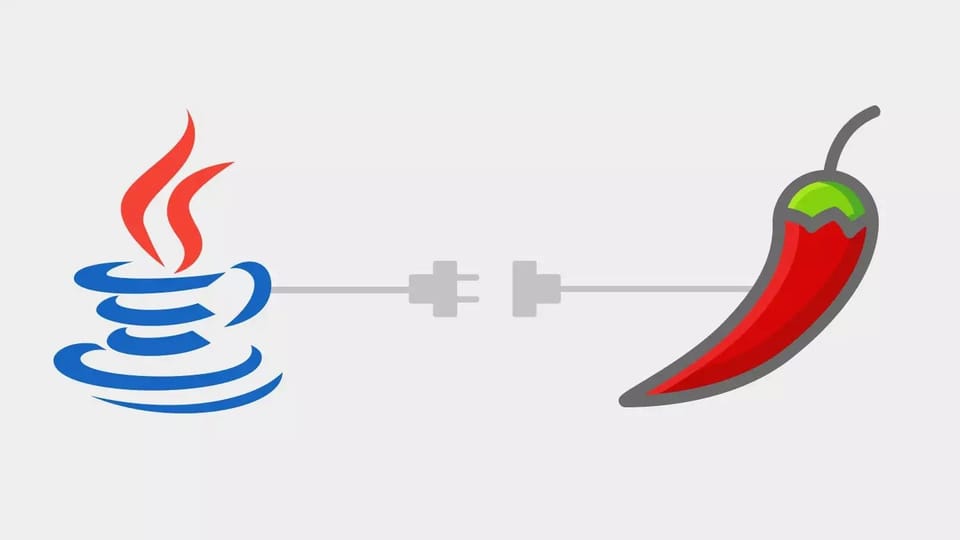
Lombok's @Getter/@Setter annotations, as the name implies, generate getter and setter methods for class fields. A default getter simply returns the field. A default setter takes 1 parameter of the same type as the field, sets the field to this value and returns void.
The generated getter/setter methods will be public unless you explicitly specify an AccessLevel (public, private, protected or package).
You can also disable getter/setter generation for any field by using the special AccessLevel.NONE.
Let's take a look at an example.
package nl.emsnsoftware.entity;
import lombok.AccessLevel;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import java.time.LocalDate;
@NoArgsConstructor()
public class Car {
@Getter @Setter
String licensePlate;
@Getter
String brand;
@Setter
LocalDate manufacturingDate;
@Getter(AccessLevel.PRIVATE) @Setter(AccessLevel.PRIVATE)
boolean inUse;
@Getter(AccessLevel.NONE) @Setter(AccessLevel.NONE)
int count;
}
By compiling the Car class, we generate the following Car.class file.
package nl.emsnsoftware.entity;
import java.time.LocalDate;
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
int count;
public Car() {
}
public String getLicensePlate() {
return this.licensePlate;
}
public void setLicensePlate(String licensePlate) {
this.licensePlate = licensePlate;
}
public String getBrand() {
return this.brand;
}
public void setManufacturingDate(LocalDate manufacturingDate) {
this.manufacturingDate = manufacturingDate;
}
private boolean isInUse() {
return this.inUse;
}
private void setInUse(boolean inUse) {
this.inUse = inUse;
}
}
Let's check each field within the generated code seperately.
The @Getter and @ Setter annotations were added to the licensePlate field. In the compiled code public methods getLicensePlate and setLicensePlate were created.
For the brand field only a @Getter annotation was added, therefore only the getBrand method was generated. Similarly for the manufacturingDate field only a @Setter annotation was added, therefore only the setManufacturingDate method was generated.
The inUse field is an exception. Since this field is of type boolean, the generated methods getter and setter method are called isInUse(instead of getInUse) and setInUse. And since we marked the annotation with AccessLevel.PRIVATE, the generated methods are marked with the private access modifier.
The last field count is marked with @Getter and @Setter annotations but with AccessLevel.NONE, therefore no getter nor setter method was generated.
Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog.