Lombok - @EqualsAndHashCode
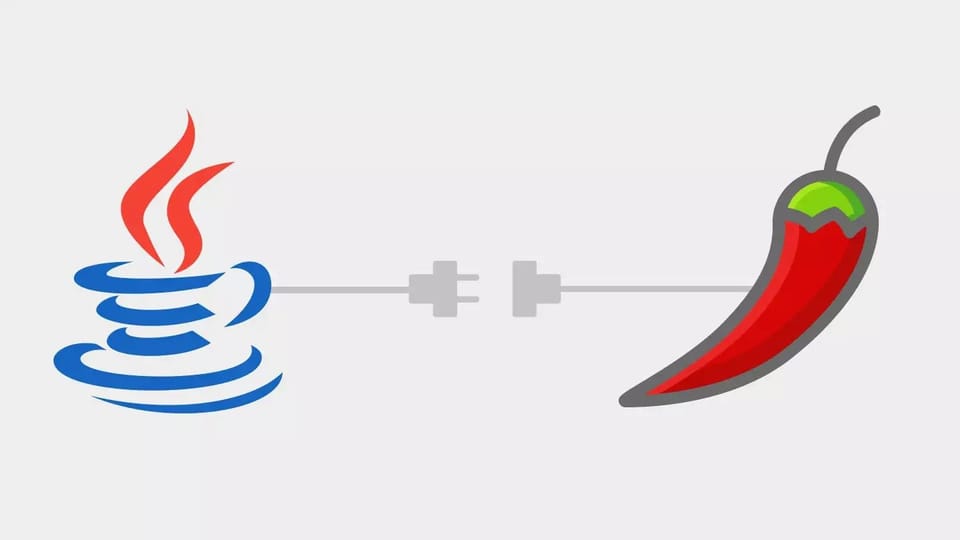
A class definition annotated with @EqualsAndHashCode lets Lombok generate the equals() and hashCode() methods implementation. By default, it'll use all non-static, non-transient fields.
You can also modify which fields are used by marking class fields with @EqualsAndHashCode.Include or @EqualsAndHashCode.Exclude. Alternatively, you can specify exactly which fields or methods you want to be used by marking them with @EqualsAndHashCode.Include and using @EqualsAndHashCode(onlyExplicitlyIncluded = true). Check out the @ToString page for more details about how to configure the generation of the method using those annotations.
Let's take a look at the following example which shows the basic usage of this annotation.
package nl.emsnsoftware.entity;
import lombok.*;
import java.time.LocalDate;
@NoArgsConstructor()
@EqualsAndHashCode
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
static int count;
}
And the compiled .class code:
package nl.emsnsoftware.entity;
import java.time.LocalDate;
public class Car {
String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
static int count;
public Car() {
}
public boolean equals(Object o) {
if (o == this) {
return true;
} else if (!(o instanceof Car)) {
return false;
} else {
Car other = (Car)o;
if (!other.canEqual(this)) {
return false;
} else if (this.inUse != other.inUse) {
return false;
} else {
label49: {
Object this$licensePlate = this.licensePlate;
Object other$licensePlate = other.licensePlate;
if (this$licensePlate == null) {
if (other$licensePlate == null) {
break label49;
}
} else if (this$licensePlate.equals(other$licensePlate)) {
break label49;
}
return false;
}
Object this$brand = this.brand;
Object other$brand = other.brand;
if (this$brand == null) {
if (other$brand != null) {
return false;
}
} else if (!this$brand.equals(other$brand)) {
return false;
}
Object this$manufacturingDate = this.manufacturingDate;
Object other$manufacturingDate = other.manufacturingDate;
if (this$manufacturingDate == null) {
if (other$manufacturingDate != null) {
return false;
}
} else if (!this$manufacturingDate.equals(other$manufacturingDate)) {
return false;
}
return true;
}
}
}
protected boolean canEqual(Object other) {
return other instanceof Car;
}
public int hashCode() {
int PRIME = true;
int result = 1;
int result = result * 59 + (this.inUse ? 79 : 97);
Object $licensePlate = this.licensePlate;
result = result * 59 + ($licensePlate == null ? 43 : $licensePlate.hashCode());
Object $brand = this.brand;
result = result * 59 + ($brand == null ? 43 : $brand.hashCode());
Object $manufacturingDate = this.manufacturingDate;
result = result * 59 + ($manufacturingDate == null ? 43 : $manufacturingDate.hashCode());
return result;
}
}
Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog.