Lombok - @Cleanup
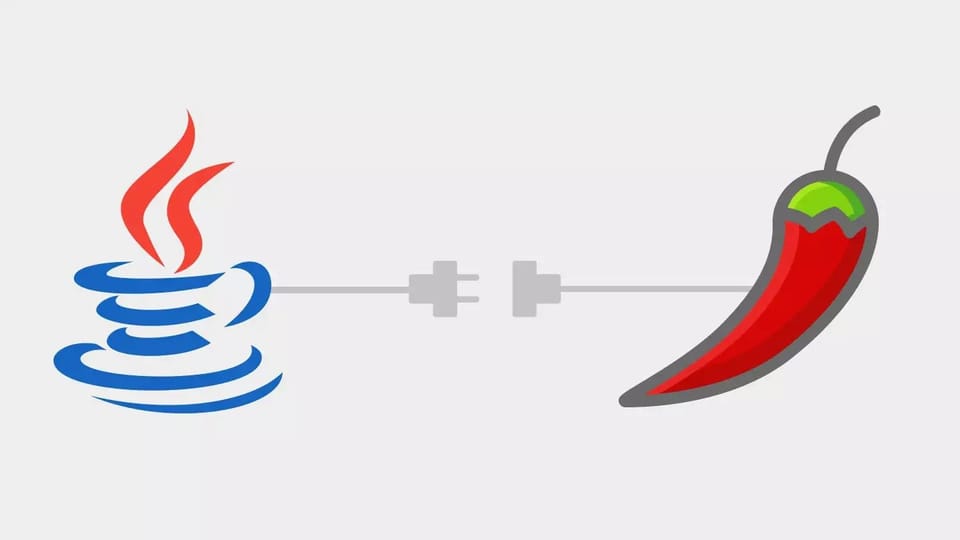
The problem of biolerplate code for resources that need to be closed and handle exceptions that might be thrown in the way is very common. The Spring framework uses the Template design patters to tackle this issue (JDBCTemplate, JmsTemplate, etc..) which moves the biolerplate code into an abstact class and implementations are responsible for the dynamic parts. But in case you want to ensure closing the open resources only then @Cleanup annotation does the job.
All you need to do is annotate any local variable declaration with the @Cleanup annotation and as a result, at the end of the scope you're in, close() will be called. Of course the declared variable has to be of a class that implements Closeable or has a close() method.
Let's take a look at an example:
package nl.emsnsoftware;
import lombok.Cleanup;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
public class App {
public static void main( String[] args ) throws IOException {
@Cleanup
InputStream inputStream = new ByteArrayInputStream(args[0].getBytes(StandardCharsets.UTF_8));
}
}
This is a very simple class that reads the first argument as an array of bytes and converts it into an inputStream. At the end of this process, you need to ensure closing this resource correctly. But you also need to check first if the resources is equal to null. Well let's see how adding @Cleanup made our life easier by checking the generated .class file below:
package nl.emsnsoftware;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.util.Collections;
public class App {
public App() {
}
public static void main(String[] args) throws IOException {
InputStream inputStream = new ByteArrayInputStream(args[0].getBytes(StandardCharsets.UTF_8));
if (Collections.singletonList(inputStream).get(0) != null) {
inputStream.close();
}
}
}
From the example we can notice that the null check and the close() method were generated for us by simply adding the @Cleanup annotation.
If the type of object you'd like to cleanup does not have a close() method, but some other no-argument method, you can specify the name of this method like so:
@Cleanup("dispose").
Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog.