Lombok - @AllArgsConstructor
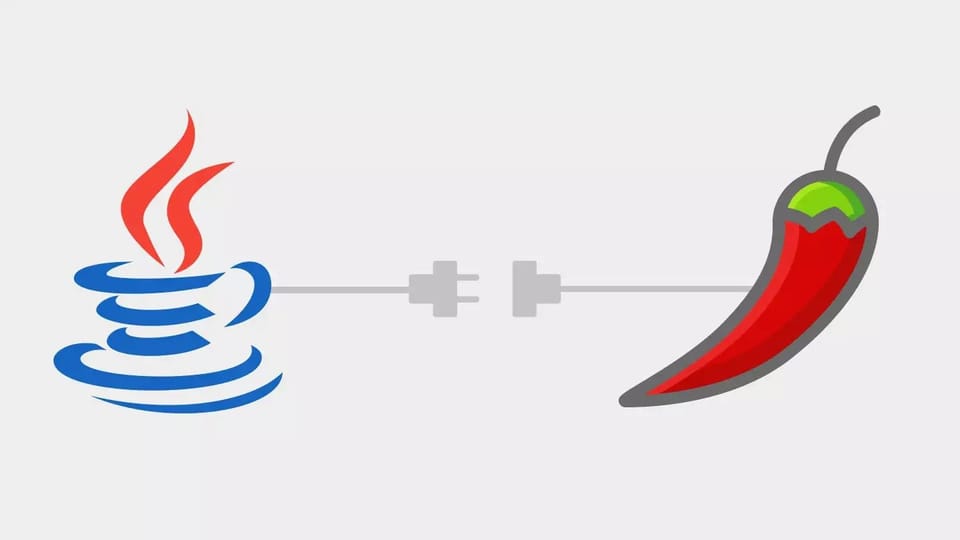
The last constructor generating annotation is the @AllArgsConstructor. The @AllArgsConstructor will generate a constructor with 1 parameter for each field in your class. Fields which are marked with @NonNull result in null checks on those parameters.
Let's take a look at the following example:
Here is the code for our Car class. What do you think the constructor will look like in the compiled code?
import lombok.AllArgsConstructor;
import lombok.NonNull;
import java.time.LocalDate;
@AllArgsConstructor
public class Car {
@NonNull
final String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
int count;
}
After compiling the code by running mvn clean install, our car.class file will look like the following:
package nl.emsnsoftware.entity;
import java.time.LocalDate;
import lombok.NonNull;
public class Car {
@NonNull
final String licensePlate;
String brand;
LocalDate manufacturingDate;
boolean inUse;
int count;
public Car(@NonNull String licensePlate, String brand, LocalDate manufacturingDate, boolean inUse, int count) {
if (licensePlate == null) {
throw new NullPointerException("licensePlate is marked non-null but is null");
} else {
this.licensePlate = licensePlate;
this.brand = brand;
this.manufacturingDate = manufacturingDate;
this.inUse = inUse;
this.count = count;
}
}
}
We can notice that a constructor with 5 parameters with generated and that corresponds with the number of instance variables of the Car class.
Lombok also generated a null check for the licensePlate field since it is marked with @NonNull annotation.
Thank you for reading my blog. Please make sure to check out the other Lombok annotations articles by visiting the Lombok section of my blog.